Ruby's .then Method: Enhancing Code Readability through Chaining Operations
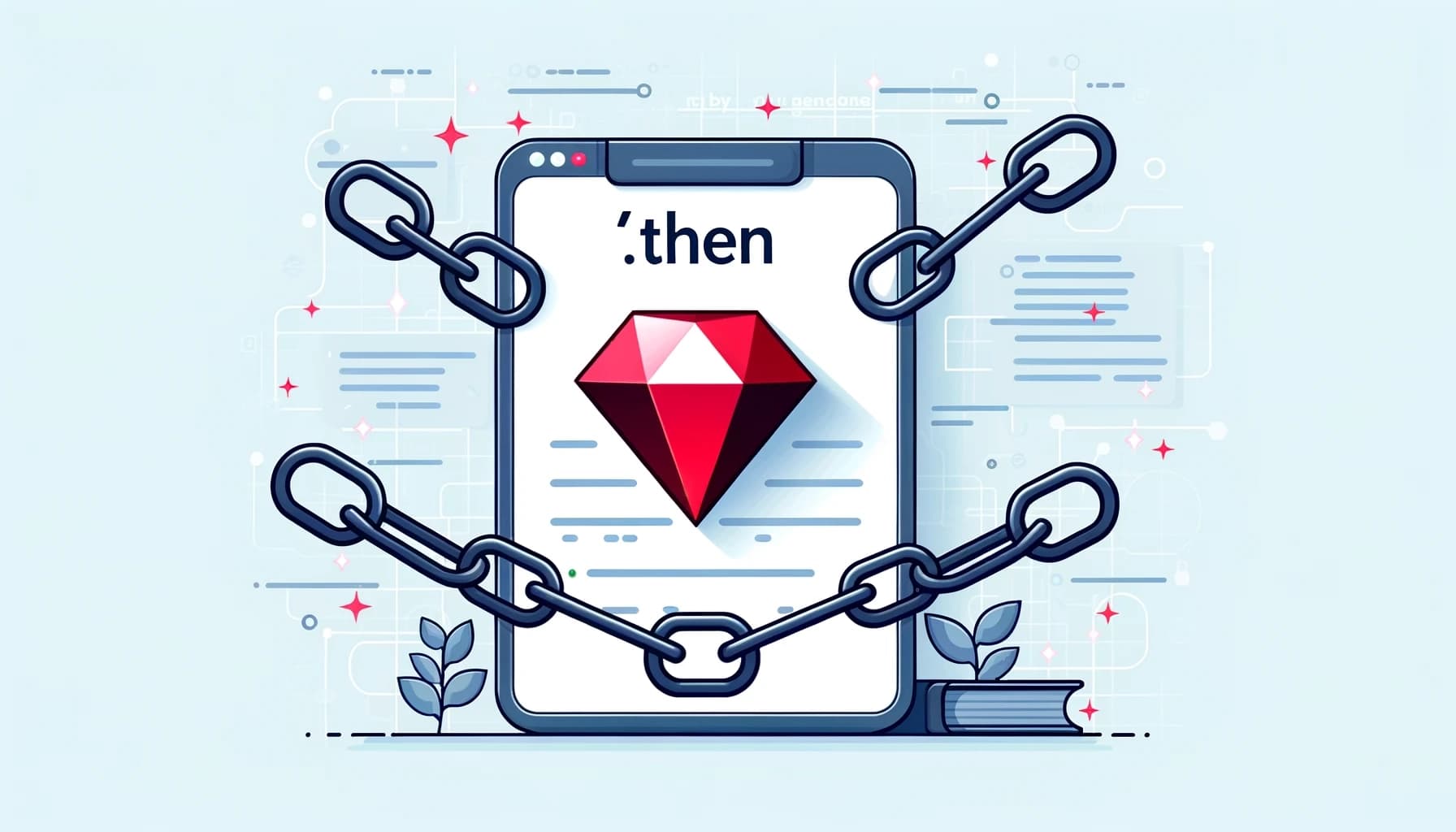
The .then
method significantly enhances the readability of chained operations. Its ability to linearly transform nested calls into a more digestible sequence of transformations is particularly beneficial in maintaining clarity in complex operations. While it might not always be the simplest solution, its contribution to code maintainability and readability makes it a valuable tool in a Ruby programmer's arsenal.
The best use of .then
is where it enhances the understanding and flow of the code, aligning with the specific needs and styles of your projects.
# chaining without .then
result = some_complex_method(another_method(a_method(value)))
# with .then
result = value.then { |v| a_method(v) }
.then { |v| another_method(v) }
.then { |v| some_complex_method(v) }
The .then
method allows the result of one expression to be passed directly into a subsequent block for further transformation, making it particularly useful in scenarios where multiple operations need to be performed in sequence.
# complex funcational oerations
parsed = parse_content(file_content)
filtered = filter_data(parsed)
enriched = enrich_data(filtered)
# with .then
file_content.then { |content| parse_content(content) }
.then { |parsed| filter_data(parsed) }
.then { |filtered| enrich_data(filtered) }
Advantages of Using .then
for Chaining
- Clarity:
.then
turns nested and potentially confusing method calls into a clear sequence of operations. - Maintainability: By breaking down operations,
.then
makes it easier to modify or extend the logic. - Functional Style: It promotes a functional programming style, reducing side effects and improving predictability.